Using Tinydancer with a Frontend App
In this example we will run the tinydancer client locally and use TS code to interact with it.
Follow the installation instructions in the Getting Started section.
Make sure the RPC server is running
The custom RPC node with merkle shreds enabled should be running and both the RPC and websocket ports should be port forwarded to your machine. Checkout the RPC Node section for more details.
Start the tinydancer client
tinydancer start "/mnt/tiny-db"
This is how the tinydancer client looks like once it is running.

Send the transaction in typescript
const txn = await signTransaction(transferTransaction);
const sendTxnPayload = {
jsonrpc: "2.0",
id: 1,
method: "sendTransaction",
params: [txn.serialize()],
};
const { data } = await axios.post("http://0.0.0.0:8890", sendTxnPayload);
Check the status of the transaction
let res = await axios.post("http://0.0.0.0:8890", {
jsonrpc: "2.0",
id: 1,
method: "getSignatureStatuses",
params: [
[data.result],
{
searchTransactionHistory: true,
},
],
});
if (!res.data.result.context.sampled) {
alert("dont trust confirmation");
} else {
alert("txn confirmed!");
}
Show the confirmation in the UI
You can create a modal to show the confirmation of the transaction.
import {
Box,
Flex,
Modal,
ModalBody,
ModalContent,
ModalOverlay,
Spinner,
Text,
} from "@chakra-ui/react";
import React from "react";
function Confirm({ isOpen, onOpen, onClose, conf1, setConf1 }: any) {
return (
<>
<Modal isOpen={isOpen} onClose={onClose}>
<ModalOverlay />
<ModalContent>
<ModalBody>
<Box h="300px">
<Flex
flexDir="column"
alignItems="center"
justify="center"
h="full"
>
{conf1 ? (
<Text fontSize="3xl">✅</Text>
) : (
<Spinner
w="4rem"
h="4rem"
color="green.500"
thickness="4px"
/>
)}
{conf1 ? (
<Text
textAlign="center"
fontFamily="Inter"
fontWeight="bold"
mt="4"
fontSize="md"
>
Transaction Confirmed! 🎉
</Text>
) : (
<Text
textAlign="center"
fontFamily="Inter"
fontWeight="bold"
mt="4"
fontSize="md"
>
Waiting for confirmation from tinydancer
</Text>
)}
</Flex>
</Box>
{/* </Flex> */}
</ModalBody>
</ModalContent>
</Modal>
</>
);
}
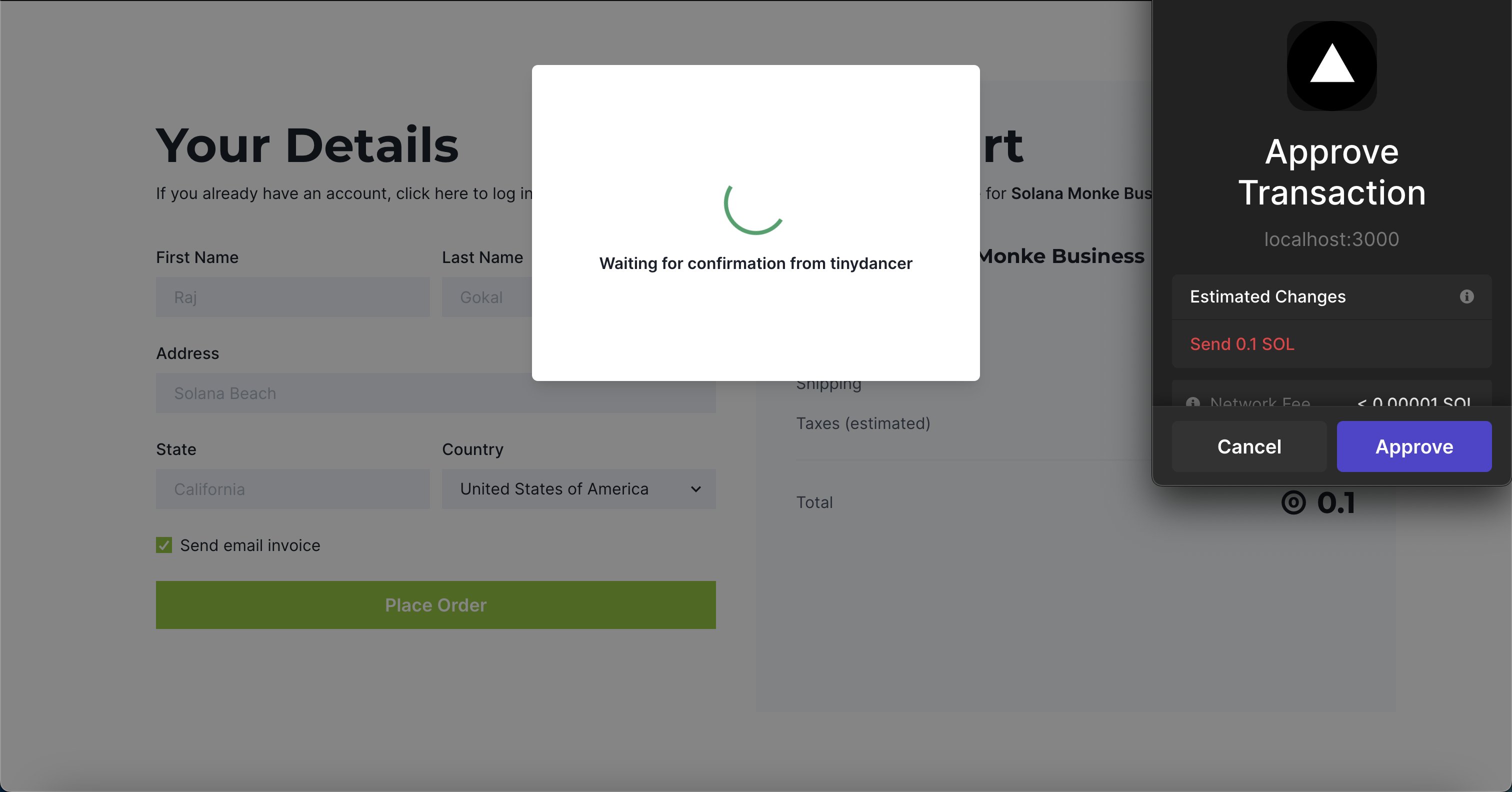
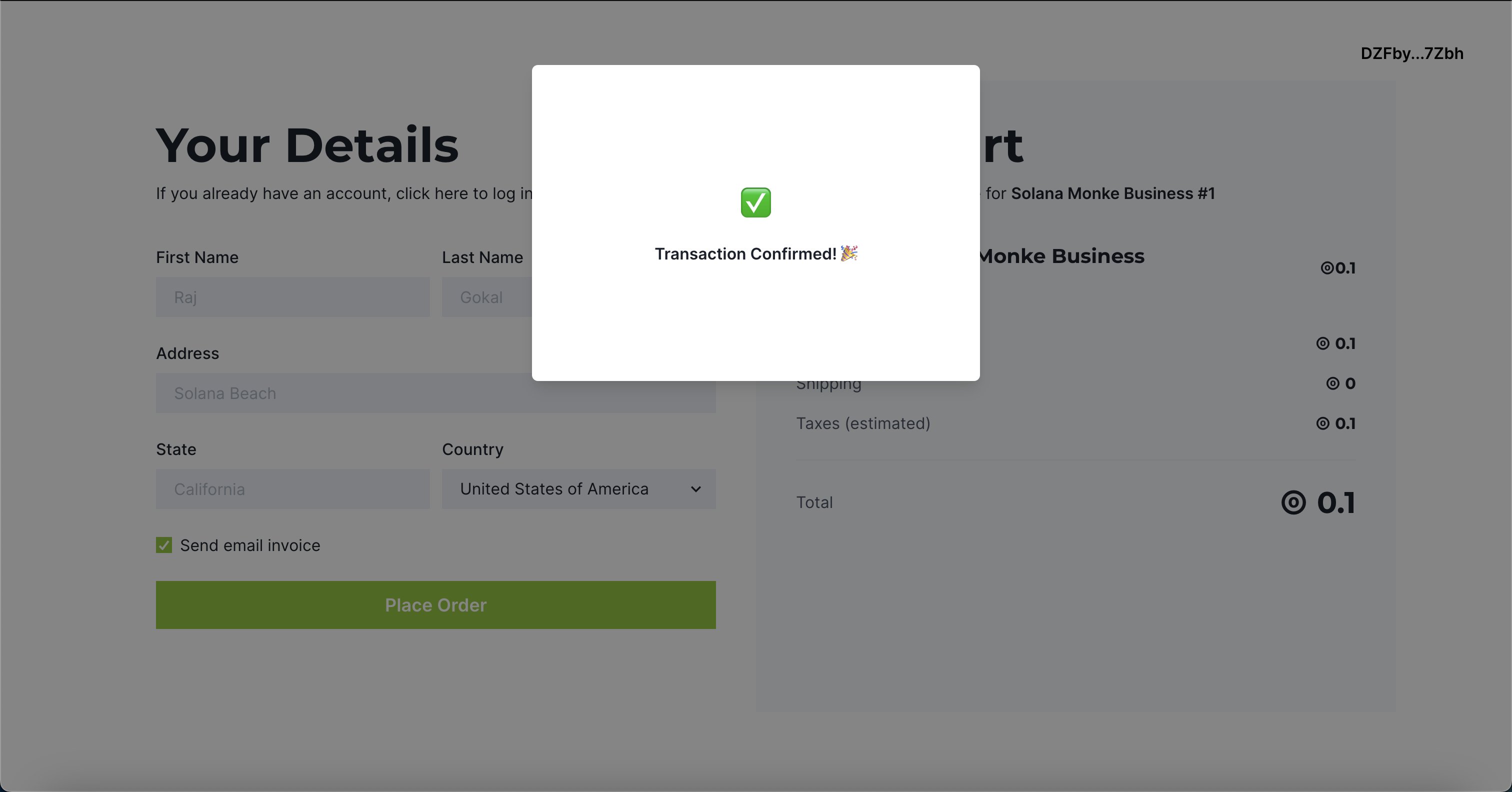
Checkout our GitHub Repo below for the example code.